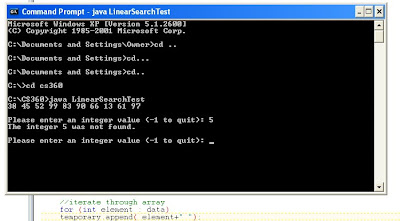
/* LinearArray */
import java.util.Random;
public class LinearArray
{
private int[] data; //array of values
private static Random generator = new Random();
// Create array of any size and fill with random int
public LinearArray( int size)
{
data = new int[size]; //creates spaces for array
//fills array with ints 10-99
for(int i=0; i
data[i] = 10 + generator.nextInt(90);
}
//perform a linear search on the data
public int linearSearch( int searchKey )
{
//loop thourgh array sequentionally
for (int index=0; index
if( data[index] == searchKey)
return index; //return inex int
return -1; //integer not found
}
//method to output values in array
public String toString()
{
StringBuilder temporary = new StringBuilder();
//iterate through array
for (int element : data)
temporary.append( element+" ");
temporary.append("\n"); //add endline
return temporary.toString();
}
}
---------------------------------------------------------------
/*LinearSearchTest*/
import java.util.Scanner;
public class LinearSearchTest
{
public static void main( String args[])
{
// create Scanner object to input data
Scanner input = new Scanner( System.in );
int searchInt; // search key
int position; // location of search key in array
// create array and output it
LinearArray searchArray = new LinearArray( 10 );
System.out.println( searchArray ); // print array
// get input from user
System.out.print(
"Please enter an integer value (-1 to quit): " );
searchInt = input.nextInt(); // read first int from user
// repeatedly input an integer; -1 terminates the program
while ( searchInt != -1 )
{
// perform linear search
position = searchArray.linearSearch( searchInt );
if ( position == -1 ) // integer was not found
System.out.println( "The integer " + searchInt +
" was not found.\n" );
// get input from user
System.out.print(
"Please enter an integer value (-1 to quit): " );
searchInt = input.nextInt(); // read next int from user
}
}
}
No comments:
Post a Comment